Search for scientific processes¶
Introduction¶
Here we demonstrate how to search for events of specific scientific processes using the Event Search subpackage included in AIDApy. First, we start with importing the modules required by Event Search:
[1]:
#Python modules
from datetime import datetime
import numpy as np
#AIDApy modules
from aidapy import event_search
---------------------------------------------------------------------------
ModuleNotFoundError Traceback (most recent call last)
<ipython-input-1-781f059ab734> in <module>
4
5 #AIDApy modules
----> 6 from aidapy import event_search
ModuleNotFoundError: No module named 'aidapy'
Looking for specific EDR events¶
In particular, here we want to look for Electron Diffusion Regions, which are tiny regions located at the very center of magnetic reconnection. To do so, we chose to use data from the MMS mission, which has been specifically designed to study the microphysics (down to electron scales) of magnetic reconnection. We also chose to look at data on 2017 July 20, during which a specific campaign was dedicated to the observation of reconnection in the magnetotail. The time interval is thus defined as:
[6]:
start_time = datetime(2017, 8, 20, 2, 0, 0)
end_time = datetime(2017, 8, 20, 2, 10, 0)
Then, we need to set up the settings for the specific scientific process we want to study; i. e., here Electron Diffusion Region (EDR). The EDR is characterized by a very low magnetic field (< 5 nT), high-speed electron jets (> 4000 km/s) and a high currents (current density > 70 nA/m²). Thus we define these criteria as inputs to the Event Search tool, in a format compliant with the event_search function (see the Event Search section in the user guide). Additionally, we chose to download data from all probes on board MMS mission, in the default GSE coordinate system. Finally, since the EDR region size is of electron scale, we chose to use the high-resolution burst-mode data from MMS, and a time window of 60 s.
[7]:
settings = {"criteria": lambda dc_mag_tot, j_curl_tot, e_bulkv_x: (np.any(dc_mag_tot < 5)) &
(np.any(j_curl_tot > 70)) & (np.any(np.abs(e_bulkv_x) > 4000)),
"parameters": {"mission": "mms",
"process": "edr",
"probes": ['1'],
"mode": "brst",
"time_window": 120,
"time_step": 120}}
Once the settings on the data and parameters are defined, we call event_search function as follows:
[9]:
event_search(settings, start_time, end_time, plot=True)
C:\Users\breuillard\AppData\Local\Continuum\anaconda3\lib\site-packages\heliopy-0+unknown-py3.7.egg\heliopy\data\util.py:601: UserWarning: The CDF provided units ('0, 1') for key 'mms1_des_compressionloss_brst' are unknown
warnings.warn(message)
C:\Users\breuillard\AppData\Local\Continuum\anaconda3\lib\site-packages\heliopy-0+unknown-py3.7.egg\heliopy\data\util.py:601: UserWarning: The CDF provided units ('0, 1') for key 'mms1_des_steptable_parity_brst' are unknown
warnings.warn(message)
C:\Users\breuillard\AppData\Local\Continuum\anaconda3\lib\site-packages\heliopy-0+unknown-py3.7.egg\heliopy\data\util.py:601: UserWarning: The CDF provided units ('00-32') for key 'mms1_des_sector_despinp_brst' are unknown
warnings.warn(message)
C:\Users\breuillard\AppData\Local\Continuum\anaconda3\lib\site-packages\heliopy-0+unknown-py3.7.egg\heliopy\data\util.py:601: UserWarning: The CDF provided units ('hours') for key 'mms1_mec_mlt' are unknown
warnings.warn(message)
C:\Users\breuillard\AppData\Local\Continuum\anaconda3\lib\site-packages\heliopy-0+unknown-py3.7.egg\heliopy\data\util.py:601: UserWarning: The CDF provided units ('hours') for key 'mms1_mec_mlt' are unknown
warnings.warn(message)
C:\Users\breuillard\AppData\Local\Continuum\anaconda3\lib\site-packages\heliopy-0+unknown-py3.7.egg\heliopy\data\util.py:601: UserWarning: The CDF provided units ('hours') for key 'mms1_mec_mlt' are unknown
warnings.warn(message)
C:\Users\breuillard\AppData\Local\Continuum\anaconda3\lib\site-packages\heliopy-0+unknown-py3.7.egg\heliopy\data\util.py:601: UserWarning: The CDF provided units ('hours') for key 'mms2_mec_mlt' are unknown
warnings.warn(message)
C:\Users\breuillard\AppData\Local\Continuum\anaconda3\lib\site-packages\heliopy-0+unknown-py3.7.egg\heliopy\data\util.py:601: UserWarning: The CDF provided units ('hours') for key 'mms3_mec_mlt' are unknown
warnings.warn(message)
C:\Users\breuillard\AppData\Local\Continuum\anaconda3\lib\site-packages\heliopy-0+unknown-py3.7.egg\heliopy\data\util.py:601: UserWarning: The CDF provided units ('hours') for key 'mms4_mec_mlt' are unknown
warnings.warn(message)
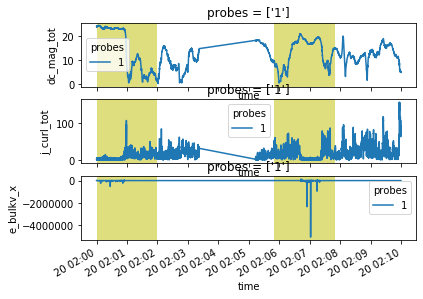
[9]:
[0, 3999, 8000, 11999]
As seen above, the function gives as ouput an overview plot with highlighted events for the user to eyeball the results, but if to_file
parameter set to True
, also writes a simple text file with the events selected, as follows:
[10]:
#events_list.txt
'''
List of events found:
N°, START TIME (UTC), END TIME (UTC), S/C location (km)
1, 2017-08-10T12:18:01.728287000, 2017-08-10T12:19:01.208817000 -96743.35/16839.555/30637.818
''';
[ ]: